Understanding and Programming Classes and Objects
In MS.NET when an object is created there is no way to get the address of an object. Only the reference to the object is given through which we can access the members of the class for a given object. When an object is created all the variables (value/ reference types) are allocated the memory in a heap as a single unit and default values are set to them based on their data types.
Account Example:
Steps to create an Account Application:
1. Create a new project (File => New Project). Name: Account Application, Project Type: C#, Template: Windows Application
2. View =>Solution Explorer, Right Click on Project => Add => Class and name it as Account
3. To class, add the following code:
using System;using System.Text;
using System.Windows.Forms;
namespace AccountApplication
{
class Account
{
public int Id;
public String Name;
public Decimal Balance;
public Account()
{
MessageBox.Show(“Object Created”);
}
~Account()
{
MessageBox.Show(“Object Destroyed”);
}
}
}
Explanation:
Ø Account a;
“a” is a reference variable of type Account.
Note: “a” is not an object of type Account.
Ø a = new Account();
Creates an object of type Account (allocating memory on the heap to every member of the Account class) and its reference is assigned to ‘a’.
Every member allocated memory is set to its default value based on the data type.
The value of the reference variable is a reference to an object on the heap.
o Account a; //Declaration
o a1 = new Account(); //Initialization
4. In the Account Application, change the name of the Form, “Form1” to “AccountForm” and design the following GUI
5. For every control on the form set Text and Name properties.
6. In Design View, double click on every button to generate event handlers in Code View of the Form
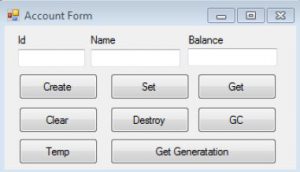
7. Add the following code to the Account Form i.e. Handle all the buttons Click event (Double click on button in design view)
using System;using System.Text;
using System.Windows.Forms;
namespace AccountApplication
{
public partial class AccountForm : Form
{
Account a;
private void btnCreate_Click(object sender, EventArgs e)
{
a = new Account();
}
private void btnGet_Click(object sender, EventArgs e)
{
txtId.Text = a.Id.ToString();
txtName.Text = a.Name;
txtBalance.Text = a.Balance.ToString();
}
private void btnClear_Click(object sender, EventArgs e)
{
txtId.Text = “”;
txtName.Text = “”;
txtBalance.Text = “”;
}
private void btnSet_Click(object sender, EventArgs e)
{
a.Id = int.Parse(txtId.Text);
a.Name = txtName.Text;
a.Balance = decimal.Parse(txtBalance.Text);
}
private void btnDestroy_Click(object sender, EventArgs e)
{
a = null;
}
private void btnGC_Click(object sender, EventArgs e)
{
GC.Collect();
}
private void btnTemp_Click(object sender, EventArgs e)
{
Account a1 = new Account();
a = a1;
}
private void btnGetgeneration_Click(object sender, EventArgs e)
{
MessageBox.Show(GC.GetGeneration(a).ToString());
}
}
}